How To Remove Duplicate Code Properly From Your App? [EXAMPLES]
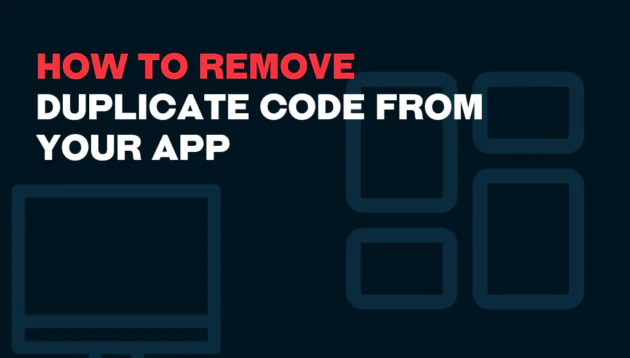
Introduction
Eliminating duplicate code is essential for maintaining a healthy codebase, reducing the risk of errors, and ensuring ease of modification of mobile and web applications. In this article, we will go with you through practical strategies on how to remove duplicate code. By using these methodologies, developers can improve the readability, maintainability, and efficiency of their applications.
The Issue of Code Duplication
Code duplication poses a significant challenge in the field of software engineering. It is a situation where identical code or very similar code fragments exist in multiple places within an application.
While it may seem like a minor issue at first glance, code duplication can lead to various complications and hindrances in the mobile or web development process.
The Role of the DRY Programming Principle
A fundamental programming principle known as DRY, which stands for “Don’t Repeat Yourself,” underscores the importance of mitigating duplicate code. The DRY principle advocates for the removal of redundancy in Java code or any other code lines by ensuring that every piece of knowledge or logic within a system is represented in a single, authoritative location. Adhering to this principle helps prevent the proliferation of duplicate code.
Why is Duplicate Code Problematic?
There are several key reasons why code duplicates are problematic:
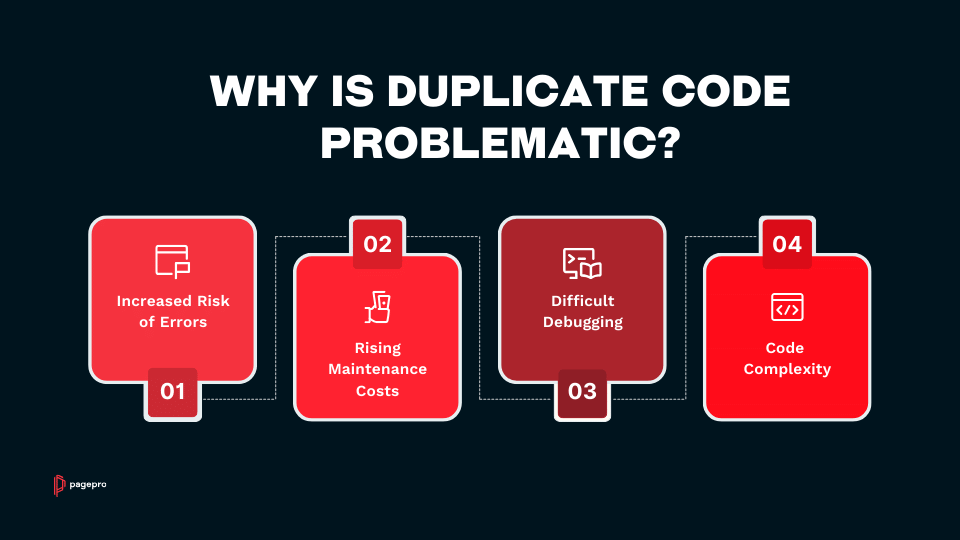
- Increased Risk of Errors: Any changes made to duplicated code fragments require updates in all their occurrences. This raises the risk of introducing errors as developers might forget one of the places where the code is duplicated.
- Rising Maintenance Costs: As a project grows, maintaining multiple copies of the same code becomes increasingly costly. Updates, fixes, and adjustments must be applied in many locations, resulting in excessive workload.
- Difficult Debugging: When a bug is found in one of the duplicated code fragments, it necessitates searching through all other instances to ensure the issue has been resolved everywhere.
- Code Complexity: The presence of code duplicates can lead to increased source code complexity, making it harder to understand and maintain the application.
Below we present live examples of how to remove duplicate code in JavaScript.
Need support in removing duplicated code?
Identifying Code Duplicates
Identifying code duplicates is a critical first step in the process of eliminating redundancy and enhancing the maintainability of your codebase. We will explore various methods and tools that can provide support and answers to fix such issues, assisting in the detection and assessment of code duplicates within your software project. By addressing these copies, developers can ensure a more streamlined and error-free development process. But first, let’s explore the ways to identify code duplicates.
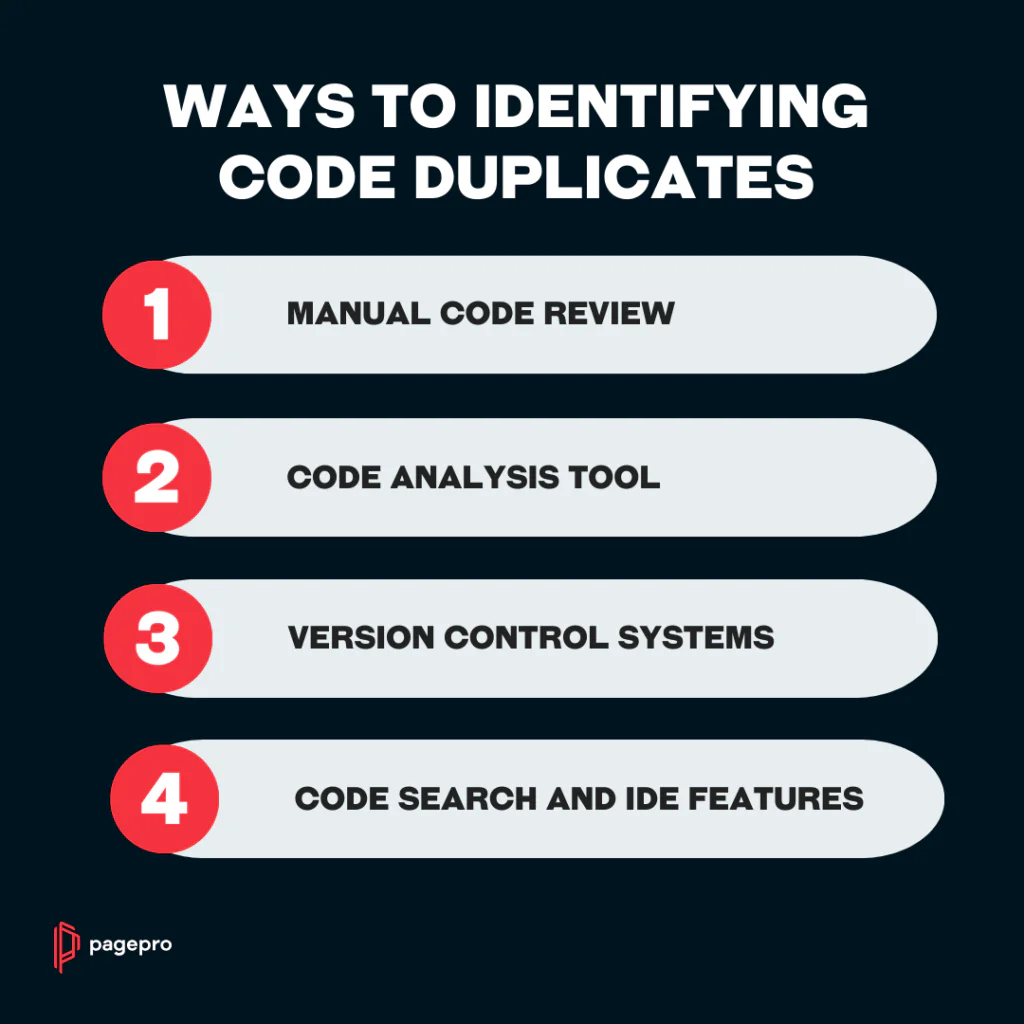
- Manual Code Review: Performing a manual code review with your development team is the answer to effectively identifying code duplicates. This collaborative effort allows team members to share their insights and personal experience in spotting redundancies.
- Code Analysis Tools: Utilizing code analysis tools and static analyzers can automate the process of identifying duplicate code. Tools such as SonarQube, PMD, and ESLint can scan your codebase and provide reports on duplicated blocks of code giving you wider context.
- Version Control Systems: Version control systems (e.g., Git) can assist in identifying duplicate code by highlighting similarities between different versions of files or code changes. Some Git extensions and plugins are designed specifically for this purpose.
- Code Search and IDE Features: Integrated Development Environments (IDEs) often come with built-in code search and analysis features. These tools can help you quickly locate duplicate code within your project.
Once you’ve identified potential duplicate codes, it’s essential to assess them to determine whether they should be eliminated or refactored. Documenting the identified duplicates, including their locations and contexts, is also crucial for a structured approach to their resolution.
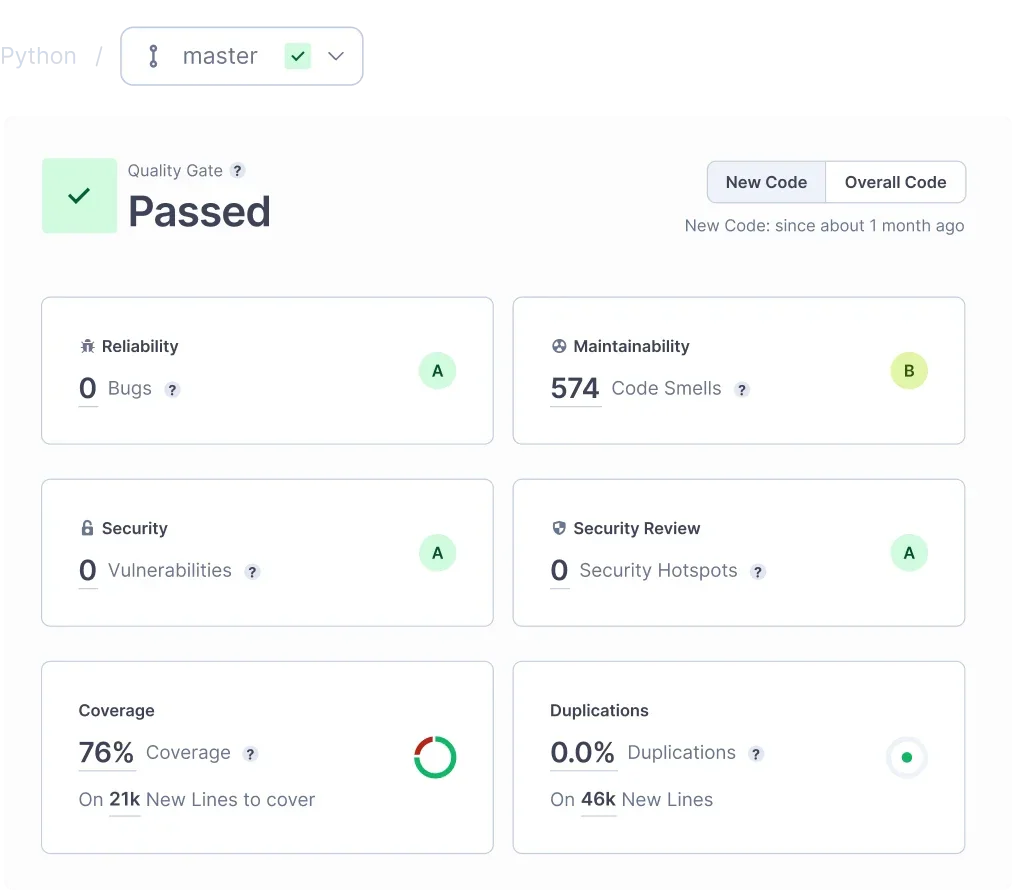
SonarQube is an excellent tool for performing static code analysis. It offers valuable assistance to your team in maintaining code cleanliness.
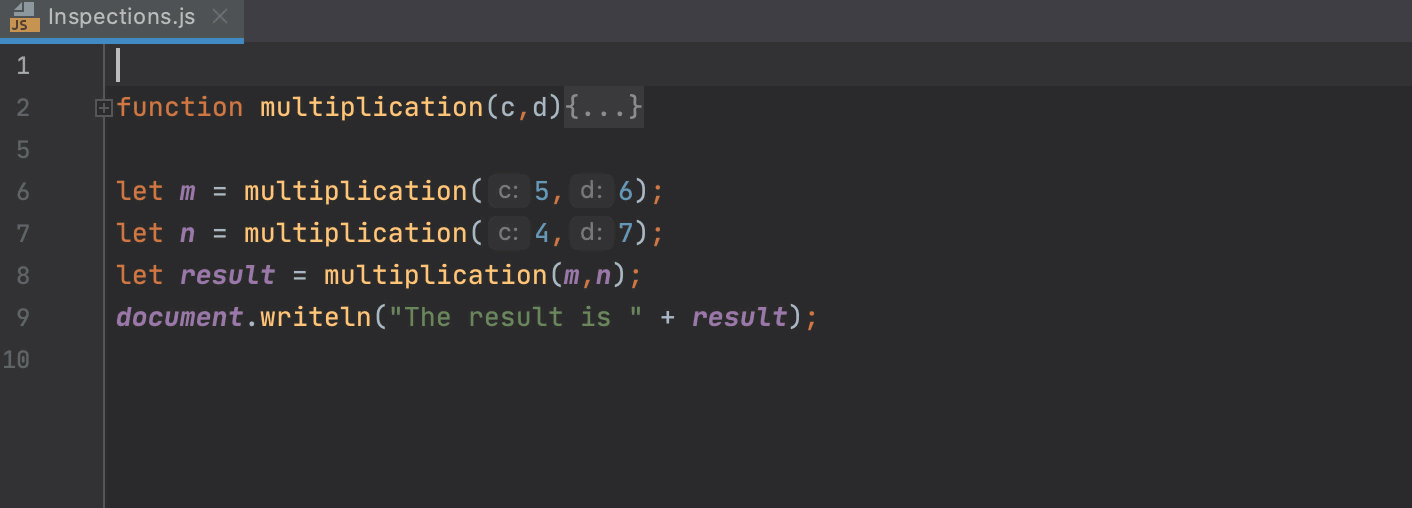
Some IDEs like Webstorm provide dedicated tools for searching and reducing code duplication.
Refactoring and Creating Reusable Functions and Classes
One of the most effective strategies for eliminating code duplication is refactoring – the process of restructuring existing code without changing its external behaviour. In this chapter, we will explore the concept of refactoring and how it can help in creating reusable functions and classes, reducing redundancy, and improving code maintainability.
Creating reusable functions to Remove duplicate code
Let’s take an example of removing duplicated code by creating a function to handle a common operation in two places:
// Duplicated code for calculating the area of a rectangle
const rectangleLength = 5;
const rectangleWidth = 3;
const rectangleArea = rectangleLength * rectangleWidth;
// Duplicated code for calculating the area of a triangle
const triangleBase = 4;
const triangleHeight = 6;
const triangleArea = (triangleBase * triangleHeight) / 2;
console.log(`Rectangle area: ${rectangleArea}`);
console.log(`Triangle area: ${triangleArea}`);
To remove the duplication, you can create a reusable function to calculate the area for both shapes:
// Reusable function to calculate the area of a shape
function calculateArea(base, height, shape) {
if (shape === 'rectangle') {
return base * height;
} else if (shape === 'triangle') {
return (base * height) / 2;
} else {
throw new Error('Unsupported shape');
}
}
// Calculate the area of a rectangle
const rectangleArea = calculateArea(5, 3, 'rectangle');
// Calculate the area of a triangle
const triangleArea = calculateArea(4, 6, 'triangle');
console.log(`Rectangle area: ${rectangleArea}`);
console.log(`Triangle area: ${triangleArea}`);
Here’s a step-by-step process to refactor and create reusable functions:
- Identify the duplicated code: Locate the sections of code that are duplicated throughout your project.
- Analyze the code: Ensure that the code can indeed be generalized into a reusable function without breaking any existing functionality.
- Create a function: Extract the duplicated code into a new function. Ensure that the function has a clear and meaningful name that describes its purpose.
- Pass parameters: If necessary, pass parameters to the function to make it flexible and adaptable to different situations.
- Test the function: Thoroughly test the newly created function to ensure it behaves as expected.
- Replace duplicates: Replace the duplicated code with calls to the new function.
Creating Reusable Classes
In addition to functions, refactoring can also involve creating reusable classes when the duplicated code involves more complex logic. Here are the steps for creating reusable classes:
- Identify duplicated logic: Locate sections of code that involve duplicated logic or algorithms.
- Extract the logic: Extract the duplicated logic into a new class. This class should encapsulate the bbehaviourand data associated with the duplicated code.
- Instantiate the class: Instantiate the new class wherever the duplicated code was previously used.
- Configure and reuse: Configure the class instances as needed, and reuse them throughout your project.
class Dog {
//Duplicated constructor
constructor(name) {
this.name = name;
}
//Duplicated move method
move() {
console.log(`${this.name} moves`);
}
voice() {
console.log('Woof woof!');
}
}
class Fish {
//Duplicated constructor
constructor(name) {
this.name = name;
}
//Duplicated move method
move() {
console.log(`${this.name} moves`);
}
voice() {
console.log('...');
}
}
We can see that constructors and move methods are duplicated in both classes. To get rid of that issue we can create a parent class named Animal to share some logic between children’s classes.
class Animal {
constructor(name) {
this.name = name;
}
move() {
console.log(`${this.name} moves`);
}
}
class Dog extends Animal {
voice() {
console.log('Woof woof!');
}
}
class Fish extends Animal {
voice() {
console.log('...');
}
}
Inheritance and Polymorphism in Object-Oriented Programming
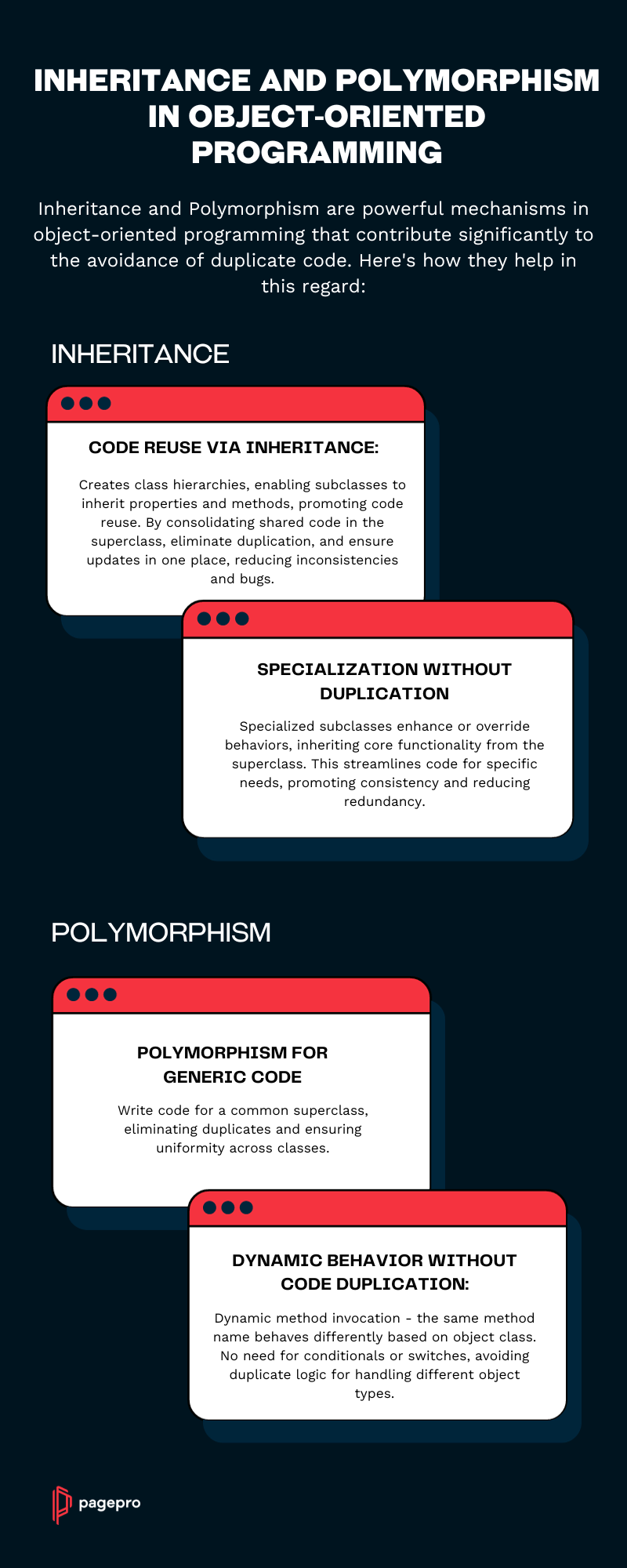
Inheritance and Polymorphism are powerful mechanisms in object-oriented programming that contribute significantly to the avoidance of duplicate code. Here’s how they help in this regard:
Code Reuse through Inheritance:
Inheritance allows you to create a class hierarchy where a subclass inherits properties and methods from a superclass. This inheritance relationship enables the reuse of code defined in the superclass across multiple subclasses. By placing common code in the superclass, you prevent the need to duplicate it in each subclass. This ensures that changes or updates to shared functionality only need to be made in one place, reducing the risk of inconsistencies and bugs.
Specialization Without Duplication:
Inheritance allows you to create specialized subclasses that can add or override behaviours specific to their needs while inheriting the general functionality from the superclass. This specialization ensures that you can adapt and extend existing code to meet specific requirements without duplicating the common logic. Consequently, you maintain consistency and reduce redundancy in your codebase.
Polymorphism for Generic Code:
Polymorphism enables you to write code that operates on objects of a common superclass without needing to know the specific subclass. This generic approach eliminates the need for duplicate code that handles similar tasks for different classes. Instead, you can write it once, targeting the common interface shared by multiple classes. This simplifies maintenance and ensures that changes or improvements apply uniformly to all relevant classes.
Dynamic Behavior without Code Duplication:
Polymorphism also facilitates dynamic method invocation, where the same method name behaves differently based on the actual class of the object. This dynamic behaviour eliminates the need for conditionals or switches to determine object types and execute the appropriate code. Consequently, you avoid duplicating conditional logic for handling various object types.
Extracting Constants and Configurations to remove duplicate code
In software development, it’s crucial to manage constants and configurations effectively. Extracting these values into separate constants or configuration files enhances code readability, maintainability, and flexibility. In this chapter, we’ll explore the importance of extracting constants and configurations, and we’ll provide practical code examples in JavaScript to demonstrate how to do it.
Why Extract Constants and Configurations?
- Readability: Constants and configurations often represent important values within your program. Extracting them into named constants or configuration files with meaningful names makes the code more self-explanatory.
- Maintainability: When constants or configurations change, you only need to update them in one place, rather than searching through your codebase for every occurrence.
- Flexibility: Separating constants and configurations allows for easy adjustment and adaptability to different environments (development, staging, production) without modifying the code itself.
Extracting Constants in JavaScript:
Consider a scenario where you have a maximum character limit for user input in multiple places across your application. Instead of hardcoding this limit, you can extract it into a constant:
// Before extraction
function validateInput(input) {
if (input.length > 100) {
return "Input exceeds the maximum character limit of 100.";
}
}
We can see that the character limit is hardcoded. This can lead to a situation where we need to edit all the occurrences of that value during specification change.
// Extract characters limit value into separate file - conts.js
export const MAX_CHARACTER_LIMIT = 100;
// Now we have a single source of characters limit in the whole project.
function validateInput(input) {
if (input.length > MAX_CHARACTER_LIMIT) {
return `Input exceeds the maximum character limit of ${MAX_CHARACTER_LIMIT}.`;
}
}
Extracting Configurations in JavaScript:
One powerful way to eliminate duplicate code in your JavaScript applications is by extracting configurations. When you have repeated values or settings in your code, extracting them into a central configuration source reduces redundancy and makes your code more maintainable. Here’s how you can achieve this:
// Duplicate code
function fetchUserData() {
return fetch('https://api.example.com/user');
}
function fetchProductData() {
return fetch('https://api.example.com/product');
}
In this example, the API URL ‘https://api.example.com’ is duplicated in two functions. To handle that we can create a configuration object to store these values in one place.
const config = {
apiUrl: 'https://api.example.com',
apiKey: 'your-api-key',
maxRetryAttempts: 3,
logLevel: 'debug',
};
Now, replace the duplicated code with the values from your configuration object.
function fetchUserData() {
return fetch(config.apiUrl + '/user');
}
function fetchProductData() {
return fetch(config.apiUrl + '/product');
}
By using config.apiUrl, you ensure that the API URL is consistent across your codebase.
Maintaining a Coding Style Guide
In the quest to eliminate duplicate code effectively from your application, one valuable tool in your arsenal is a well-maintained coding style guide. A coding style guide promotes code consistency and enforces best practices that inherently discourage code duplication.
At its core, a coding style guide is a complete document that codifies coding conventions, standards, and recommended practices to be adhered to by developers when writing code. While it encompasses a broad spectrum of topics, its impact on the removal of duplicate code is profound. Here’s why:
Consistency Matters: A coding style guide enforces consistency in code structure, formatting, and naming conventions. Consistent code is easier to read and scrutinize for duplicated patterns.
Readable Code Aids Detection: Well-structured, readable code is a foundation for code duplication identification. When code is clear and structured according to the guide, spotting duplicate logic becomes more apparent.
Modularity Encouragement: Many coding style guides advocate for modularity. This approach encourages developers to break down complex logic into reusable functions or modules, reducing the chances of code repetition.
Conclusion
The battle against code duplication is a fundamental quest for cleaner, more maintainable, and efficient applications. This article explained why and how to remove duplicate code properly from your app, emphasizing the critical importance of this endeavour.
The journey began by recognizing that code duplication and identifying and acknowledging duplicated logic is the first step toward better code.
We explored a series of strategies and best practices:
- Identifying Code Duplicates: Recognizing and acknowledging duplicated code is the crucial first step in our journey to cleaner software.
- Refactoring and Creating Reusable Functions and Classes: Extracting and organizing code into reusable functions and classes streamlines our codebase for better maintenance.
- Extracting Constants and Configurations: Centralizing constants and configurations improves readability and adaptability while reducing redundancy in our code.
- Maintaining a Coding Style Guide: A well-maintained coding style guide emerged as a cornerstone in promoting code consistency and discouraging duplication.
Throughout this journey, we learned how to remove duplicate code and that it is an ongoing commitment to code quality. Regular code reviews, refactoring processes, and diligent documentation all contribute to this continuous improvement process.
Need support in removing duplicated code?
Read more
18 tips for a better code review
A Quick Guide for React Native Version Updates